If you’ve wondered how websites delay animations until elements are in view, the Intersection Observer API is the tool for the job. It allows you to trigger actions, like animations, when elements enter or exit the viewport.
Here’s how it works
In the example below the class “visible” is being added to the animate-on-scroll class when the user is scrolling into the viewport where the animate-on-scroll is located and then removed when user is scrolling pass it.
The rootMargin is an offset applied to the root element’s bounding box, expanding or shrinking the area in which the observer checks for intersections.
The threshold defines how much of the target element must be visible before the observer’s callback is triggered. It’s a value between 0 and 1
Pretty cool, right.
const observer = new IntersectionObserver((entries) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
entry.target.classList.add('visible');
} else {
entry.target.classList.remove('visible');
}
});
}, {
rootMargin: '0px 0px -50px 0px', // Adjust this margin to your needs
threshold: 0.3
});
document.querySelectorAll('.animate-on-scroll').forEach(element => {
observer.observe(element);
});
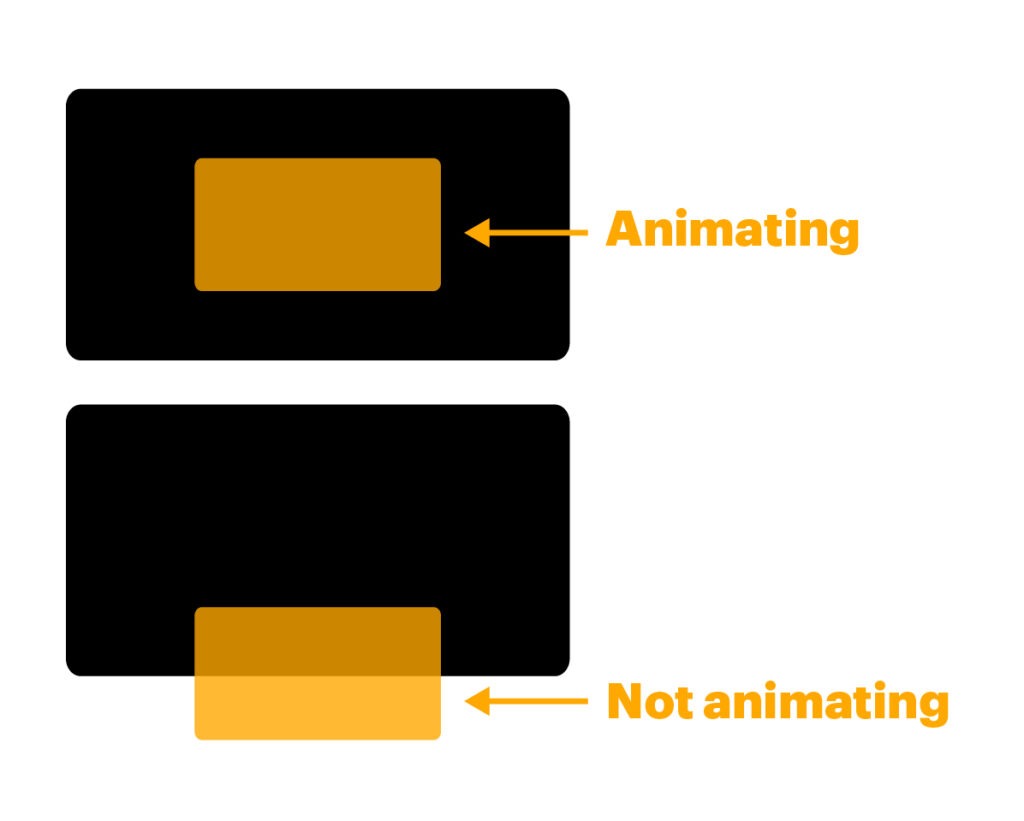
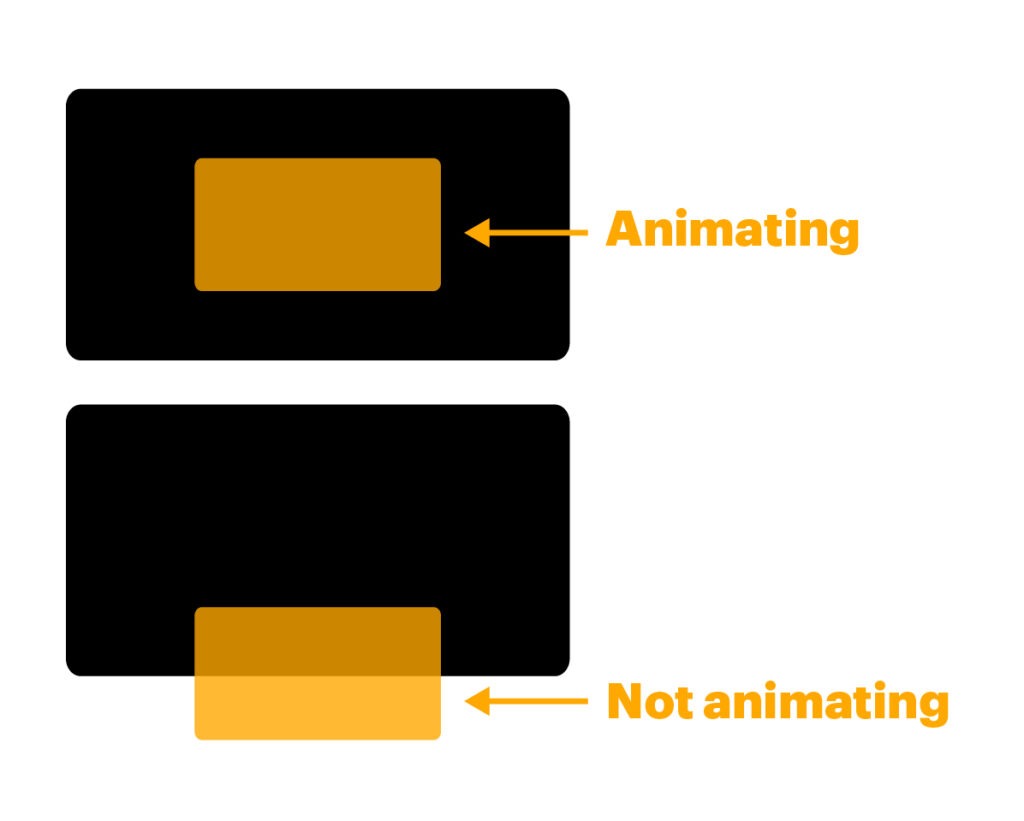
The Intersection Observer kicks in when the element is in the viewport (the threshold can be set to kick it off sooner or later then 100%),